First 30 days of development
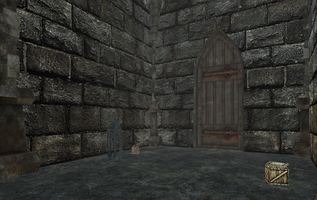
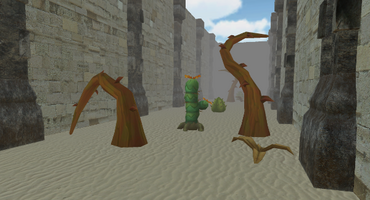
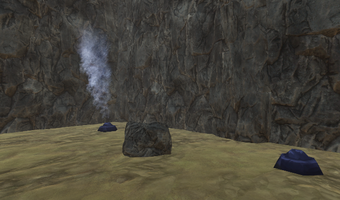
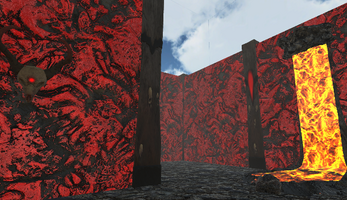
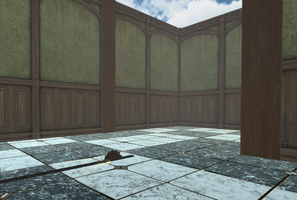
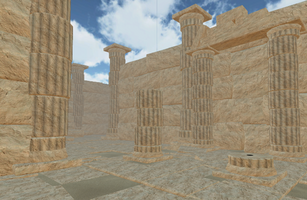
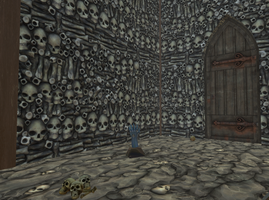
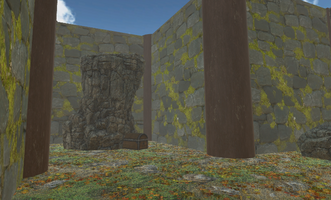
(This is a long post - tldr: I've been making a game...)
I have been developing Labyring for just over 30 days now, here is a synopsis of what has happend during that time:
Day 1:
This whole adventure started with a programming challenge I set for myself. I wanted to write a program that could generate a maze.
That wasn't too hard, after a few hours I had a maze generator up and running, I even made it solve the mazes for me. (you can download the Maze Generator here)
Day 2:
I had a thought; what if I could walk around in the mazes?
I spent most of the day writing a unity script that could read in the mazes that the maze generator makes and create a level based on that:
The Walls and ground all come from the Dungeon Castle pack by InfinityPBR
The DungeonGeneration Script works by taking in a sprite generated by the the MazeGenerator (generated by anything really). You set the path color (Yellow in the above image) and the paze Multiplier. The multiplier is a size (in pixels in the generator, in units in unity) that basizelly tells how wide the path is. I use 10.
Once the generator knows this, you click Generate, it then looks at every 10x10 square (Mult x Mult) and checks the edges to see if they are white or pathColor. If they are not white (or yellow) it knows to put a wall there. In reality, the maze only checks the left and bottom sides of each maze chunk. It also places floors.
Pillars are placed at every intersection. this was done to keep the walls from flickering when you walked by. The pillars are slightly bigger so they can hide this ugliness.
Foreshadowing: Keep in mind, the maze knows the path to the goal! Each floor knows if it isPath.
Day 3:
Wandering around a huge maze wasn't very exciting, this is when I came up with a plan to make a real game.
I thought of adding monsters and equipment and fighting and death!!! But I decided to start simple, lets get the maze to dynamically add chests and doors and stuff:
The Chests come from the Mimic Chests pack by InfinityPBR
This works actually by changing the Maze Generator. The generator finds the correct path by following ALL dead ends. (google Dead-end filling algorithm). I used this to my advantage, The program loops through the maze finding all dead ends and filling them. Well, I have the maze put a dot in each dead end in the FIRST loop. This marks every real dead end. Then in the DungeonGeneration script, I check for that dot in each square and place a chest if I find it.
Day 4:
Adding chests wasn't too difficult, but finding/opening chests is boring too, what if I had to pick open the chests with lockpicks!
So I spent all of the 4th day getting a lockpicking system working:
The Lockpicking stuff came from the Lock-picking pack by InfinityPBR. This took a bit of work to do. Being a former locksmith, and knowing ALOT about how locks work, I had to make this work properly. It took a bit of modification to make everything perfect, but I'm real happy with the outcome :)
Day 5:
Whats the point in having a maze with chests without a bad guy, so I spent the day making a bad guy that can chase me and attack me
The Spider resource came from InfinityPBR (you can get it on the asset store) as did ALL my monsters, InfinityPBR makes hella awesome assets. And I used Opsives Behavior Designer to control the bugger. Generally I HATE big assets (I have tried several over the years), they are too complicated and generally don't do what I want any easier than me making it from scratch, but I have to say, the Behavior Designer is super easy to get up and going, especially if you are already familiar with NavMesh.
Day 6:
Now, whats the point in having a maze with chests and bad guys if you can't fight it right?!?
I bought Opsives character controller and ended up just using the unity essentials basic one. This is one of those times when big assets are dumb. it was WAY over done and complicated for my needs. I made the animations for the sword myself, but the sword came from the Weapons and Armor pack by InfinityPBR. Yeah, I know, everything comes from InfinityPBR, but I have been subscribed to them for years and purchasing assets with the intention of using them one day... so... I'm going to put EVERY monster of theirs I have in this game ;)
Now that I have a monter, I have the DungeonGeneration script put either a monter or a chest at each dead end.
Day 7:
Fighting monsters is cool, but you really want them to fight back. So today I built a healt/stamina system aswell as a knockback system... and a shield:
Again, the Shield came from the Weapons and Armor pack by InfinityPBR. I had to make the shield animation myself because I am using a stock controller. Honestly, I'm no animator, but it's not hard to make simple animations like this. The Health and stamina bars I made using Photoshop. I am no artist, but making a a rounded cornered box is pretty easy. I made 1 asset, then in game, I made 6 copies in the UI. the back most copy is translucent(one for stamina and one for health). Then, ontop of that I place a second copy that slowly decreases using LERP on the fill % from the left. This give it the impression of decreasing health/stamina. Then finally the 3rd copy I placed on top of that, it reflects the actual value of your health/stamina and it colored red/green.
All of this had to be controlled by a UI script. The UI script just watches the player and adjusts the health/stamina as the player takes damage. The player has a Health and Stamina script that controls their current values, the UI script simply displays it.
Day 8:
Swords and shields are great, but how do we change them?
For this to work, I made an abstract class enteractable. This class can be inherited by ANYTHING the player can interact with. currently, we have chests and weapons. When the player interacts with comthing it fires the Enteract() method, regardless of what object it is. Generalized code is nice.
I also added gold and ore as stuff you get from the chests... these will be used in the various stores later.
Day 9:
Gotta be able to heal right...
This was alot of fun. I needed a way to heal the player without an inventory system, so I made a bed. The player can interact with the bed (see another enteractible) and it would start a chat script (instead of the weapon equip script above). This script can be used for anything that has yes or no questions.
Again, Opsive and Pixel Crushers both have dialogue systems I have purchased... but they are way to complciated and big. Mine is simple. Everything that can chat inherits from a chatter script. Chatter includes some basic stuff that interacts with the UI. There is also a ChatMessage object that contains text to be displayed and if it is a question. The ChatMessage also knows the index of the next message, if it is yes OR if the player answers no (only if it is a question). if the next index is ever -1, it knows to stop chatting. This means that you can make a ChatMessage[] and fill it with all the ChatMessages you want. All the Yes or No questions you want, and just direct the answers to more conversation. It's simple and it works.
Day 10:
I took a day off to play boardgames and snuggle... so here is a cat picture:
Day 11:
If the only things in the dungeon were monsters and chests, it would get pretty boring. So I added some special rooms:
Whaddayaknow, another chatter ;)
Assets in theses special rooms came from many places: Old Furniture by Diana, Mini Fantasy Pack by Robot Skeleton, Rug Pack by Azerillo, Books by Vis Games, more Old Books by PointlessClouds, and of course, Elf Character from InfinityPBR
Remember back on day 2 when I had the floor know if it was part of the path or not? This lady is the reason. She sets a timer on the player that lets him see glowing balls for the path. Granted, if you are nowhere near the path, you might waste the 500 gold... also, you could pick the wrong direction and head back away from the goal... but the code works ;)
Day 12:
Made a shop:
Being able to spend that gold is always nice. This guy is a chatter too but he has a special UI he calls, kinda like the weapons call their special Equip UI, he gets a Shop UI. He sells weapons based on how much interaction you have had with him (all shops share this value). So buy and sell stuff to be able to buy more stuff.
I also realized that the game is using too many resources when I generate a large map, so I had to write this SphereOfInfluince script that turns off objects that are too far away.
Day 13:
What was the ore for?
This guy uses the Store UI aswell, but he sets a flag to useOre. Once the flag is set, it will look at the player BluePrint list. If the player has blueprints, we can make them. Blueprints are simple objects that hold an item prefab... they are found in chests.
The human assets come from InfinityPBR (Suprised?) Also used Old Furniture from Maria K and Midieval Furniture from Alexander Voevodov... and Library Books from Andrei Selyanin
Day 14:
Today, I felt like the game was pretty playable. We've got weapons, monters, a store... all the good stuff... So I banged out a title screeen, death, pause, and loading screen... then released a demo...
BUT you can't play it... it's been too long and Demo2 is already out so too bad.
Oh, right before the demo dropped I added more to the UI. I added visible weapon and armor stats (you will see it in the next video)
Day 18:
I took the long weekend to hang with the family and came back today to make a Biome system:
The Biome System works pretty simply. You (the developer) set a size for each biome, this is in a Mult x Mult grid. For the image above, a 20x20 map, the biome size is 10. The game creates a Biome array, this is an int[] that just has values from 1-7(inclusive) that tell the game which biome to place. Now as the DungeonGenerator is making the dungeon, before it places an object in the square, it checks which biome it is in based on som rather confusing math. Then it pulls a wall/floor/pillar/monster from the BiomeList that you(the developer) have provided it. I made 8 total biomes. The center is surrounded by a special biome (0) that has no monsters. This lets the player get geared up before getting attacked.
I started to fill out the biomes a bit. I want the dungeons to have various objects in them so the player has something to look at as they go. I used Lava & Volcano Environment from NatureManurfacture to make nice lava flows in the Hell Biome. This turned out to be a problem for the spider monsters:
I created an immunity system to monsters in hell would be immune to fire damage (elemental damage has been in the game since attack boxes, spiders are resistant to poison, use poison attacks and are weak to... yeah, fire). So ontop of Weaness and resistance, we now have Immunities. A monster with an elemental immunity would take no damage from various elemental attacks. Pro Tip: Don't take a fire sword into the Hell Biome...
Day 19:
I spent the day adding stuff to more of the biomes. Made another environment trap too ;)
The Zombie Hand came from the Halloween Cemetery Set from 3dfancy, the wall/floor materials in the Catecomb biome came from Skull & Bones Textures from R33k.
The Zombie hand is not animated. I, not having any idea how to add bones to a model, went to Maximos free skeleton creator thingy... The problem is, Maximos thing is designed for humanoids... so this hand has like feet and arms and legs... I made its arms go down the thumb and pinky finger so it the animation has his hands up over his head and basically, bends over... the idle break animation is more like doing an over head forward press... but its good enough for now.
Day 20:
I finished ALL 8 biomes today. Got pretty stuff in each one:
Day 21 was spent doing other things... no update. Spent about an hour updating the spider AI
Day 22:
It's time to get more monsters... starting with the Mimic (since it's already imported)
Day 23:
The ever important Deamoness (now the spiders done die, cause she spawns in the Hell biome instead)
The deamoness came from the Deamoness pack from InfinityPBR
She has several different skins that she can spawn with and her spikes, facial features and body all mesh morph randomly when she spawns so each deamoness will look different.
She has a unique behavior compared to all the other monsters. All her attacks are fairly far away from her body, so she actually backs away from the character if you get too close.
Day 24:
Cobra Snake Monsters!!! These actually went in the Forest and Desert biomes (pullin double duty)
They came from the Cobra Snake Pack from InfinityPBR. They mesh morph too and have many skin patterns :)
The snakes have an interesting behavior. They basically sleep until you get too close to them. then they go into attack mode and chase/attack. so if you don't go near them, you can ignore them.
Also put in skeletons:
These baddies have random armor pieces added to them. each one adds 0.5 armor to their defenses. They mesh morph and have 4 different bone colors aswell.
They came from the Skeleton pack by InfinityPBR.
Day 25:
Got some general monster creatures in today:
These Monsters are generic Monster Creature 1 and Monster Creature 2 from InfinityPBR.
Both these guys mesh morph and have skin textures too. I have a script I write for each monster that decides how to randomize them. It makes sure nothing funny happens when they are randomized (Ill talk more about it later)
This actually finished, putting 1 monster in each biome that belonged there, not just spiders.
Day 26:
I added Scorpions, Ghost Knights and Goblins to the game. (all from InfinityPBR)
The goblin female clothes had me messed up for hours. So, the goblin asset has male and female bodies inside it. So my RandomGoblinEquipper script first chooses if it is a male or female. Then, The goblins have basic rag clothes and armor, the 2 don't work together. My randomizer chooses if we are using the clothes or the armor. then it equips them randomly and then meshmorphs the character.
BUT sometimes:
The goblins fleshy bits popped through the clothing. Now, this is mostly my fault, I randomly set the characters mesh morph values from -100 to 100. So anything can happen, BUT the clothing is supposed to be bigger than the body nomatter what I do... So I wrote a little post ranzomizer method that adjusts iffy values. So when I find them, I just add some fixes into the BlendShapeChecks() method and it will fix them after the randomizing is done. In this case, I manually set her Nipple size to -100 (noone will notice anyway). this makes sure it never busts through the shirt. (later, the Medusa character needed ALOT more fixes to stay decent, so I am glad I made this function)
The fruits of my hard labor:
Day 27:
I actually finished all the forest monsters (there will be 5 monsters per biome):
Day 28:
I Got Devils and Deamonsinto the Hell Biome (both from InfinityPBR):
Day 29:
Took the day off to play Divinity Original Sin 2 with the kids... so here is a cat pic:
Day 30:
The long awaited end of the first month... I reworked how monsters and walls are randomized, reated a Randomize abstract method that keeps basic functions for everything, like if it will randomize on start or not aswell as the Unity Editor button that lets me randomzie in the editor... just basic house keeping. I also sped up level generation a little.
If you enjoyed this and want to play the game, here is a demo.
In the demo there is 1 monster in each biome, 7 swords, 5 shields and all 8 biomes. There is only 1 medium sized maze to play. The game can generate random mazes at start. Small 20x20 and Medium 50x50 mazes will be infiniate, but not in a demo. In the Full release there will be 1 HUGE Labyerinth (100x100). This is where the main game will happen. There will be lore and secrets to find, a bit of a story too.
I hope you enjoyed this far too detailed write up of what I did every day for the first 30 days of development. If you like it, leave a comment. I will make weekly update like this if there is interest.
Labyrinth
A 'Historically Accurate' Maze Game
Status | In development |
Author | Locksmitharmy |
Tags | maze |
Comments
Log in with itch.io to leave a comment.
For a labyrinth, I can't wait to see the minotaur in the middle :D